How to Use the Google Search Console API in Node.js
By RG team · 20 min read
Last Updated on July 16, 2023
Wait a minute...
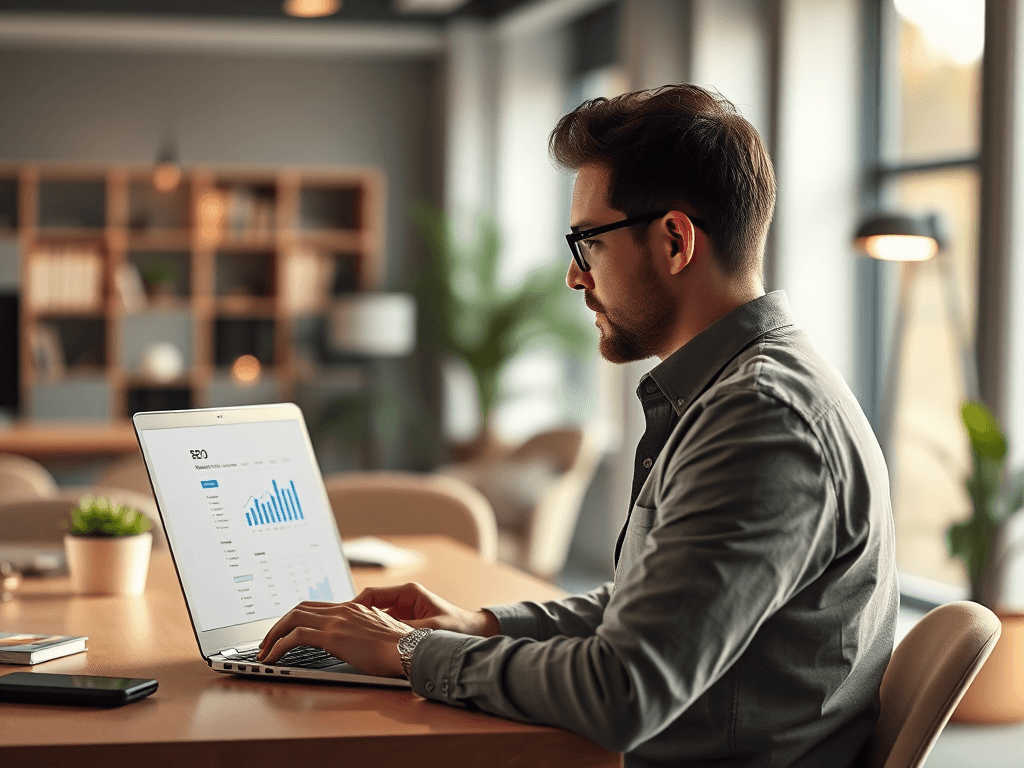
How to Use the Google Search Console API in Node.js 2023
Introduction
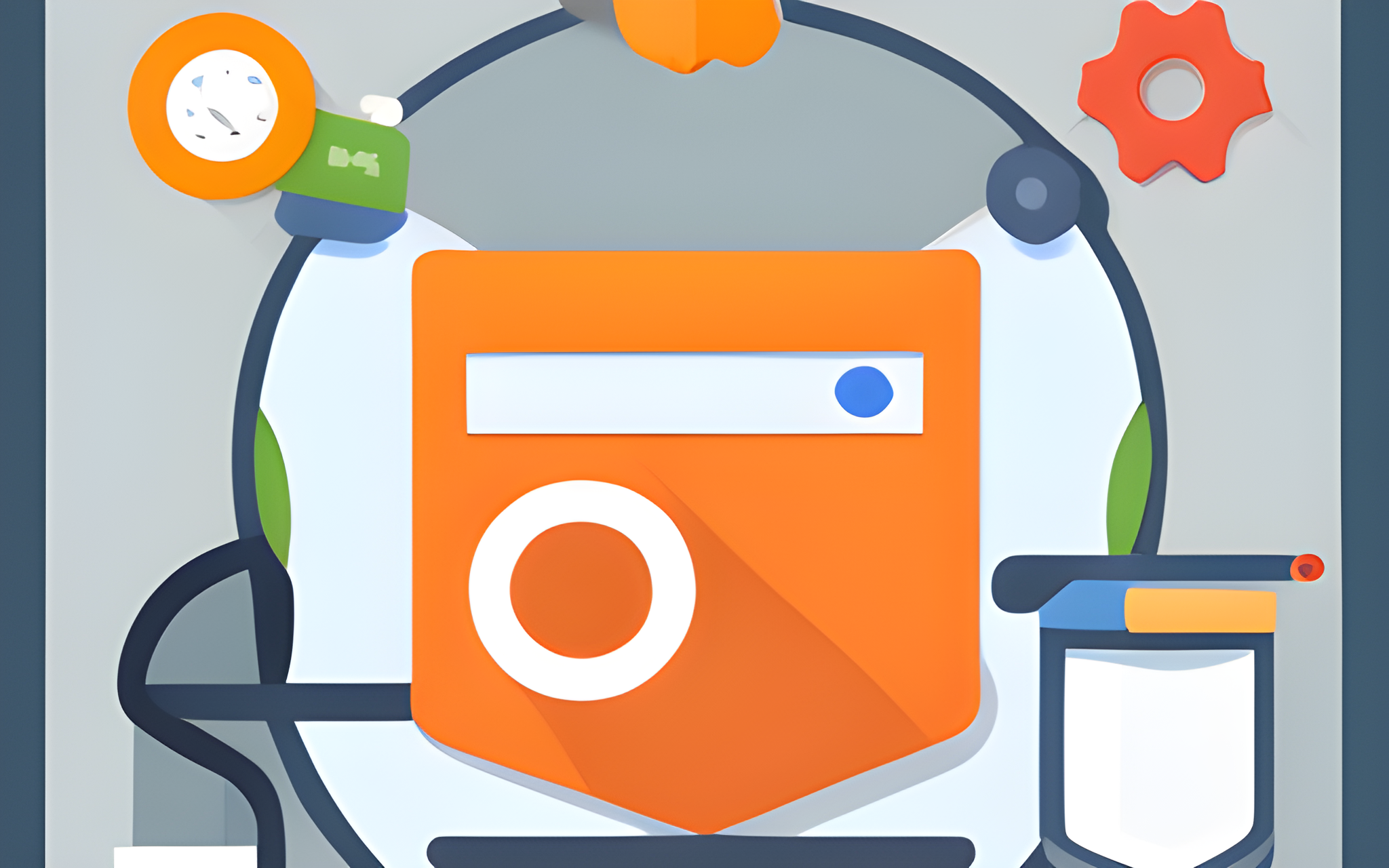
Welcome, entrepreneurs, to the exciting world of using the Google Search Console API in Node.js! In this article, we will embark on a journey to unlock the power of the Google Search Console API and discover how it can supercharge your SEO strategies. But first, let's understand what the Google Search Console API is and why it's essential for your business.
The Google Search Console API provides programmatic access to valuable reports and actions within your Search Console account, offering insights into search performance, site indexing, mobile usability, and much more. By utilizing this API in combination with Node.js, you can automate data retrieval, analyze trends, and optimize your website's SEO to drive organic traffic and boost business growth.
But why is this relevant to you as an entrepreneur? Well, every successful business relies on a solid online presence, and organic search traffic plays a pivotal role in attracting potential customers. By leveraging the Google Search Console API in Node.js, you can gain a competitive edge by staying ahead of emerging trends, uncovering low-competition, high-volume keywords, and optimizing your website for improved visibility in search engine results.
In this article, we will provide you with a step-by-step guide for utilizing the Google Search Console API in Node.js. From connecting to the API to accessing data and integrating with other tools, we will cover everything you need to know to enhance your SEO strategy. So, tighten your seatbelts, entrepreneurs, as we embark on this exciting adventure of using the Google Search Console API in Node.js!
Steps to Connect to the Google Search Console API in Node.js
Now that you understand the importance of the Google Search Console API and its potential for boosting your website's SEO, let's dive into the step-by-step process of connecting to the API in Node.js. By following these instructions, you'll be well on your way to harnessing the power of the search console data.
Step 1: Create a Project in Google Developers Console
To begin, you need to create a project in the Google Developers Console. Sign in to your Google account and navigate to the Developers Console. Once there, click on the "Create Project" button and give your project a meaningful name.
Step 2: Enable the Search Console API
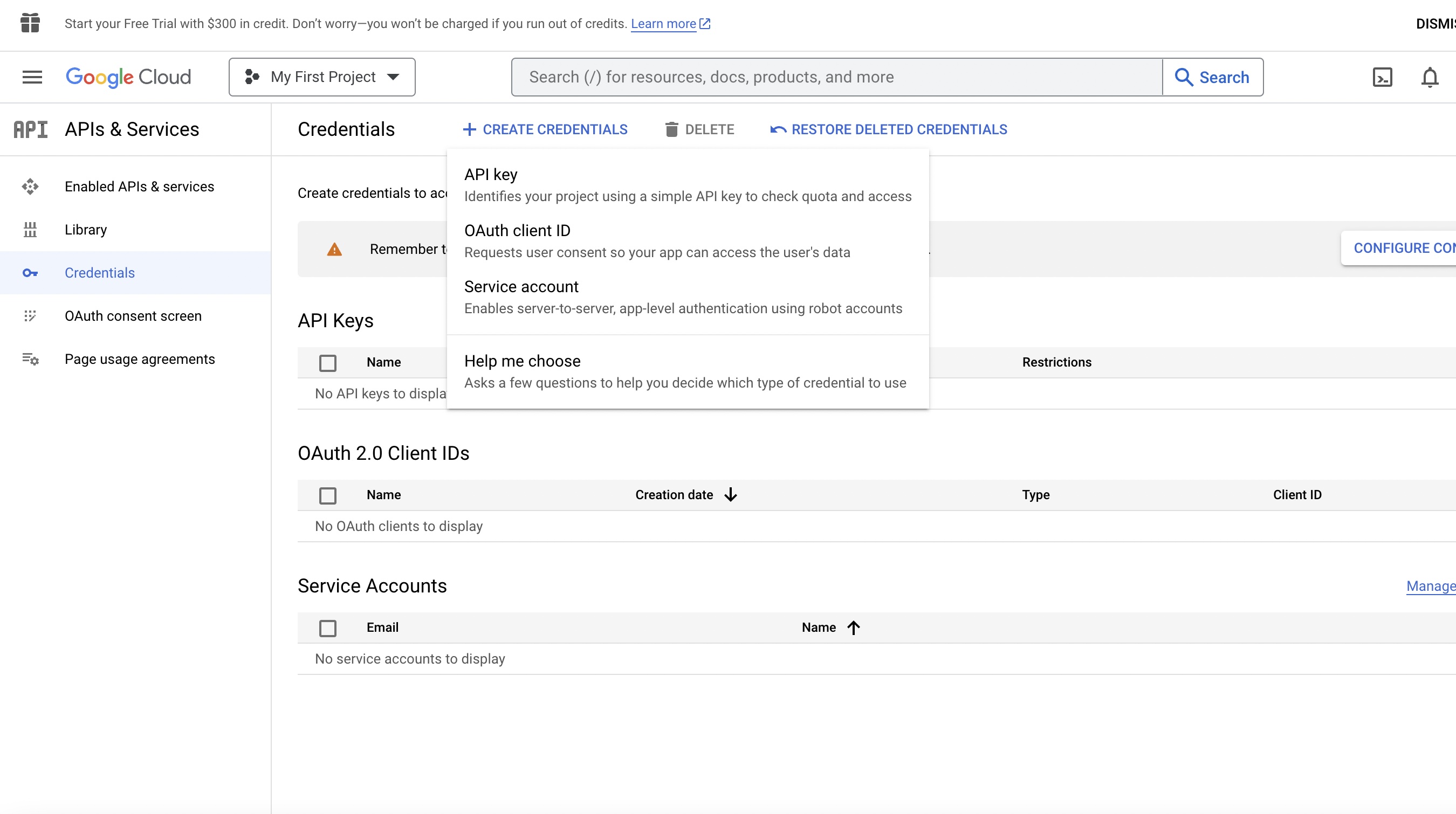
With your project created, it's time to enable the Search Console API. In the Developers Console, locate the "APIs & Services" section and click on "Library." Search for the "Search Console API" and click on it. Then, click the "Enable" button to activate the API for your project.
Step 3: Set Up Authentication Credentials
To access the Search Console API, you'll need to set up authentication credentials. In the Developers Console, navigate to the "Credentials" section, and click on "Create Credentials." Select "OAuth client ID" as the credential type, and configure the consent screen with the necessary information.
Next, you'll need to set up the authorized JavaScript origins and redirect URIs. These values determine which URLs are allowed to make requests to your API. Set the JavaScript origin to "http://localhost:3000" for development purposes, and add additional origins as needed. For the redirect URI, enter "http://localhost:3000/oauth2callback" for the default Node.js development server.
Step 4: Obtain the API Keys
Once you have set up the authentication credentials, you can obtain the API keys required for connecting to the Search Console API in your Node.js application. In the Developers Console, go to the "Credentials" section and locate your OAuth 2.0 client ID. Click on the client ID to view the details, and copy the "Client ID" and "Client Secret" values. These keys will be used in your Node.js code for authentication.
Congratulations! You have now successfully connected to the Google Search Console API in Node.js. In the next section, we will explore the key features and capabilities of the API that will empower you to optimize your website's SEO effectively. So, let's move on to the exciting part - understanding what the Search Console API has to offer!
Overview of the Key Features and Capabilities of the Google Search Console API
Now that we have successfully connected to the Google Search Console API in Node.js, it's time to explore its key features and capabilities. Understanding what the Search Console API can do will enable you to make the most of its offerings and optimize your website's SEO effectively.
1. Querying Search Analytics
One of the powerful features of the Search Console API is the ability to retrieve search analytics data. With just a few lines of code, you can access valuable insights into impressions, clicks, and average position of your website's pages in search results. This data can help you identify trends, optimize your content, and improve your website's visibility in search engine rankings.
2. Managing Sitemaps
The Search Console API also allows you to manage your website's sitemaps programmatically. You can submit new sitemaps, retrieve information about existing sitemaps, and even remove outdated or invalid ones. This feature ensures that search engines have up-to-date information about your website's structure and content, improving its indexing and visibility.
3. Fetching URL Crawl Errors
Identifying and fixing crawl errors is crucial for maintaining a healthy website. The Search Console API enables you to retrieve crawl error data, including URLs with 404 errors, server errors, or blocked content. By addressing these issues promptly, you can ensure that search engines can access and index your webpages effectively.
4. Analyzing Mobile Usability
In today's mobile-centric world, optimizing your website for mobile usability is paramount. The Search Console API provides access to mobile usability data, allowing you to identify and fix issues that may affect the user experience on mobile devices. By ensuring that your website is mobile-friendly, you can improve your search rankings and attract more organic traffic.
5. Monitoring Performance and Indexing
With the Search Console API, you can monitor the performance of your website in search results and keep track of index coverage. This includes tracking the number of indexed pages, detecting any indexing issues, and receiving notifications about important changes or observations. By staying on top of your website's performance and indexing, you can take proactive steps to optimize its visibility and reach.
Now that you have an overview of the key features and capabilities of the Google Search Console API, it's time to dive deeper into each of these functionalities and understand how to leverage them effectively in your Node.js application. In the next section, we will explore how to access and retrieve data from the Search Console API using Node.js. So, let's continue our journey of mastering the Google Search Console API in Node.js!
How to Access and Retrieve Data from the Google Search Console API in Node.js
Now that we have familiarized ourselves with the key features and capabilities of the Google Search Console API, let's delve into the process of accessing and retrieving data from the API using Node.js. By following the steps outlined in this section, you'll be able to harness the power of the API to gather valuable insights and optimize your website's SEO.
Step 1: Set Up the Google APIs Client Library
To interact with the Search Console API in your Node.js application, you'll need to install and set up the Google APIs client library. Open your terminal and navigate to your project directory. Then, run the following command to install the library:
npm install googleapis
Once the installation is complete, you can require the library in your Node.js code by adding the following line:
const { google } = require('googleapis');
Step 2: Authenticate with the API
Before making any API requests, you need to authenticate your application using the credentials obtained in the previous steps. You can configure authentication by creating a new OAuth2
client and passing in your client ID and client secret:
const oauth2Client = new google.auth.OAuth2(
'YOUR_CLIENT_ID',
'YOUR_CLIENT_SECRET',
'YOUR_REDIRECT_URL'
);
Replace 'YOUR_CLIENT_ID'
, 'YOUR_CLIENT_SECRET'
, and 'YOUR_REDIRECT_URL'
with your actual values.
Step 3: Authorize the Client
To authorize the client and obtain an access token, you'll need to generate an authorization URL and redirect the user to it. Use the generateAuthUrl
method of the OAuth2
client to get the authorization URL:
const authUrl = oauth2Client.generateAuthUrl({
access_type: 'offline',
scope: ['https://www.googleapis.com/auth/webmasters.readonly']
});
The access_type
parameter is set to 'offline'
to obtain a refresh token, which allows your application to access the API on behalf of the user without requiring their presence.
Step 4: Retrieve Authorization Code
Once the user grants permission to your application, they will be redirected back to your specified redirect URL, along with an authorization code in the query string. Capture this authorization code and store it for the next step.
Step 5: Get Access Token
Now, exchange the authorization code for an access token by calling the getToken
method of the OAuth2
client:
const { tokens } = await oauth2Client.getToken(authorizationCode);
The getToken
method will return a promise that resolves to an object containing the access token, refresh token, and other information. Store the access token securely, as it will be used to make API requests.
Congratulations! You have successfully authenticated and obtained an access token to interact with the Google Search Console API in Node.js.
Examples of Practical Use Cases and Applications of the Google Search Console API in Node.js
Now that we have successfully authenticated with the Google Search Console API in Node.js, let's explore some practical use cases and applications of this powerful tool. By understanding these examples, you'll be able to leverage the API's functionalities to optimize your website's SEO effectively.
1. Analyzing Search Performance
One valuable use case of the Search Console API is analyzing search performance data. With the API, you can retrieve metrics such as impressions, clicks, and average position for specific pages, queries, or countries. By analyzing this data, you can identify top-performing pages, high-potential keywords, and areas for improvement. This insight can guide your content strategy and help you tailor your website to the preferences and needs of your target audience.
const response = await webmasters.searchanalytics.query({
siteUrl: 'YOUR_WEBSITE_URL',
requestBody: {
startDate: 'YYYY-MM-DD',
endDate: 'YYYY-MM-DD',
dimensions: ['page', 'query'],
rowLimit: 10,
}
});
Replace 'YOUR_WEBSITE_URL'
with the URL of your website and specify the desired start and end dates for the search analytics data.
2. Monitoring Indexing Status
Another valuable application of the Search Console API is monitoring the indexing status of your website's pages. You can retrieve information about indexed pages, crawl errors, and submit new or updated sitemaps. This information enables you to ensure that search engines are indexing your pages correctly and address any issues that may hinder crawling and indexing.
const response = await webmasters.sitemaps.list({
siteUrl: 'YOUR_WEBSITE_URL'
});
Replace 'YOUR_WEBSITE_URL'
with the URL of your website to retrieve a list of sitemaps associated with your site.
3. Identifying Mobile Usability Issues
Mobile usability is crucial for attracting and retaining visitors. With the Search Console API, you can retrieve mobile usability data for your website. This includes information about mobile-friendly pages, mobile usability errors, and mobile page loading issues. By rectifying any mobile usability issues, you can enhance the user experience on mobile devices and improve your search rankings.
const response = await webmasters.mobileUsability.get({
siteUrl: 'YOUR_WEBSITE_URL',
});
Replace 'YOUR_WEBSITE_URL'
with the URL of your website to retrieve mobile usability data.
These are just a few examples of the practical use cases and applications of the Google Search Console API in Node.js. By leveraging the API's capabilities, you can gain valuable insights, optimize your website's performance, and enhance its visibility in search results. In the next section, we will explore best practices and tips for maximizing the benefits of the Search Console API in Node.js. So, let's continue our journey towards SEO excellence!
Best Practices and Tips for Optimizing SEO Using the Google Search Console API in Node.js
Now that we have explored the practical use cases and applications of the Google Search Console API in Node.js, let's dive into some best practices and tips to maximize the benefits of this powerful tool for optimizing your website's SEO.
1. Regularly Monitor and Analyze Your Data
Make it a habit to regularly monitor and analyze the data retrieved from the Search Console API. This will help you identify trends, spot issues, and make data-driven decisions to improve your website's SEO performance. Set up automated reporting and alerts to keep track of important metrics and receive notifications when there are significant changes.
2. Focus on Performance Metrics
Pay close attention to performance metrics such as impressions, clicks, and average position. These metrics provide valuable insights into how well your website is performing in search results. Identify pages with high impressions but low clicks, as they may require optimization to encourage higher click-through rates. Monitor the average position of your pages and strive to improve rankings by optimizing content and targeting relevant keywords.
3. Optimize Website Structure and Indexing
Ensure that your website's structure is search engine-friendly by submitting accurate and up-to-date sitemaps to the Search Console API. Monitor index coverage regularly to identify and address any indexing issues. Keep track of crawl errors and promptly fix them to ensure that search engines can access and index your pages effectively.
4. Mobile Optimization is Key
Given the importance of mobile usability in search rankings, pay special attention to mobile optimization. Use the mobile usability data retrieved from the Search Console API to identify and fix any issues that may affect the user experience on mobile devices. Optimize page loading speed, ensure responsive design, and provide a seamless mobile browsing experience to attract and retain mobile users.
5. Leverage Search Analytics Insights
The Search Console API provides valuable search analytics data, including top queries, popular pages, and geographic distribution of impressions and clicks. Use this information to identify high-performing keywords, optimize content for relevant queries, and tailor your website to the preferences of your target audience.
By following these best practices and tips, you can fully leverage the capabilities of the Google Search Console API in Node.js to optimize your website's SEO. In the next section, we will explore how to integrate the Search Console API with other tools and platforms to enhance your SEO strategy even further. So, let's continue our journey towards SEO excellence and discover the power of integration!
How to Integrate the Google Search Console API with Other Tools and Platforms in Node.js
To take your SEO strategy to the next level, it's essential to integrate the Google Search Console API with other tools and platforms. This integration allows you to combine the power of the Search Console API with additional functionalities, enabling you to supercharge your SEO efforts. In this section, we will explore how to integrate the Search Console API with other tools and platforms in Node.js.
1. Connect the Search Console API with Google Analytics
By integrating the Search Console API with Google Analytics, you can access a wealth of additional data and insights. Combining information about search queries, impressions, and clicks with user behavior metrics from Google Analytics provides a holistic view of your website's performance. This integration allows you to identify user trends, track conversion rates, and make data-driven decisions to optimize your SEO strategy.
2. Automate Reporting with Data Visualization Tools
To make the most of your search console data, consider integrating the Search Console API with data visualization tools such as Google Data Studio or Tableau. These tools allow you to create visually appealing and interactive reports that make it easier to understand and communicate SEO performance. With automated data retrieval and visualization, you can save time and present actionable insights to stakeholders.
3. Incorporate SEO Recommendations from SEO Tools
Integrating the Search Console API with popular SEO tools such as Moz, SEMrush, or Ahrefs can provide additional SEO recommendations and insights. These tools can analyze your website's data from the Search Console API and offer suggestions for improving keyword targeting, on-page optimization, and link building. By combining the power of multiple SEO tools, you can fine-tune your SEO strategy and stay ahead of the competition.
4. Build Custom Dashboards and Alerts
By integrating the Search Console API with dashboarding tools like Grafana or custom-built dashboards, you can create tailored visualizations and monitor key SEO metrics at a glance. Set up custom alerts to receive notifications when specific metrics deviate from desired thresholds. This integration empowers you to proactively identify issues, take timely action, and stay on top of your SEO performance.
5. Incorporate Search Console Data into Content Management Systems
Integrating the Search Console API with popular content management systems (CMS) such as WordPress or Drupal can enhance content optimization and performance tracking. By automatically fetching data from the Search Console API, you can gain real-time insights into the performance of your published content. This integration enables you to optimize existing content, identify content gaps, and streamline your content creation process.
By integrating the Google Search Console API with other tools and platforms, you can unlock a whole new level of SEO optimization. The ability to combine data, automate reporting, and gain actionable insights provides a significant advantage in the competitive online landscape. In the next section, we will discuss future trends and developments in the Google Search Console API ecosystem for Node.js, so stay tuned for exciting possibilities on the horizon!
Future Trends and Developments in the Google Search Console API Ecosystem for Node.js
As technology continuously evolves, the Google Search Console API ecosystem for Node.js also evolves to meet the changing needs of SEO professionals and website owners. In this section, we will discuss some of the future trends and developments to look out for, keeping you informed about the exciting possibilities on the horizon.
1. Enhanced Data Visualization and Insights
Google has been continuously improving its data visualization capabilities within the Search Console API. We can expect to see even more advanced and interactive data visualizations that provide deeper insights into search performance, user behavior, and website optimization. These enhancements will enable SEO professionals to analyze data more efficiently and make data-driven decisions to improve their websites' visibility.
2. Integration with Artificial Intelligence and Machine Learning
Artificial Intelligence (AI) and Machine Learning (ML) are becoming increasingly crucial in the field of SEO. Google is likely to incorporate AI and ML technologies into the Search Console API ecosystem, allowing SEO professionals to leverage advanced algorithms for keyword analysis, content optimization, and website auditing. These advancements can provide more accurate recommendations and insights, helping users stay ahead of the ever-changing SEO landscape.
3. Expansion of Mobile SEO Features
With the rising dominance of mobile devices, Google places significant emphasis on mobile usability and performance. In the future, we can anticipate an expansion of mobile SEO features within the Search Console API ecosystem. This may include enhanced mobile usability analysis, mobile-first indexing insights, and recommendations for improving mobile website performance. Optimizing for mobile will remain a critical aspect of SEO, and the Search Console API will continue to support website owners in this endeavor.
4. Integration with Voice Search and Virtual Assistants
As voice search and virtual assistants gain popularity, the Search Console API is likely to evolve to accommodate these emerging trends. We can expect features that provide insights into voice search queries, optimize content for voice-based searches, and enhance website accessibility for virtual assistant interactions. Integrating voice search data and insights into the Search Console API ecosystem will enable website owners to adapt their SEO strategies for the voice-driven future.
5. Improved Performance and Accessibility
Google consistently strives to enhance the performance and accessibility of its APIs. We can expect future developments within the Search Console API ecosystem to improve data retrieval speed, increase API limits, and provide more accessible documentation. These improvements will empower SEO professionals to work efficiently with large datasets, perform real-time analysis, and access the data they need quickly and easily.
With these future trends and developments in the Google Search Console API ecosystem for Node.js, we can look forward to even more powerful tools and capabilities for optimizing our websites' SEO. Staying up-to-date with the evolving SEO landscape and embracing these advancements will ensure that you remain competitive and achieve long-term success. In the final section, we will conclude our journey through the Google Search Console API in Node.js, summarizing the key takeaways and emphasizing its potential for driving organic traffic and business growth. So, let's proceed to our conclusion and reflect on the valuable insights we've gained!
Conclusion: Harnessing the Power of the Google Search Console API in Node.js
Throughout this article, we have explored the steps to connect to the API, its key features and capabilities, practical use cases and applications, best practices for optimization, integration with other tools and platforms, and future trends to watch out for. Armed with this knowledge, you are well-equipped to supercharge your website's SEO and drive organic traffic for business growth.
By leveraging the Google Search Console API in Node.js, you have the ability to access valuable search analytics data, manage sitemaps, monitor mobile usability, analyze performance metrics, and much more. With each feature and capability, you unlock new insights and opportunities for optimizing your SEO strategy. Remember to regularly monitor and analyze your data, focus on performance metrics, optimize website structure and mobile usability, and integrate with other tools and platforms to maximize the impact of the Search Console API.
As you continue on your entrepreneurial journey, keep in mind the importance of SEO in building a strong online presence. Organic search traffic can be a game-changer for attracting potential customers and growing your business. By harnessing the power of the Google Search Console API in Node.js, you gain a competitive advantage in the digital landscape.
Stay curious and adaptable, as the future of the Search Console API ecosystem holds even more exciting possibilities. Be prepared to embrace enhanced data visualization, AI and ML integration, mobile SEO advancements, voice search optimization, and improved performance and accessibility.
Now, it's time to put your newfound knowledge into action. Connect to the Google Search Console API, analyze your website's performance, uncover insights, and optimize your SEO strategy to achieve your entrepreneurial goals. Remember, SEO is not a one-time task but an ongoing journey of continuous improvement.
As we conclude this article, we hope that you feel empowered to navigate the ever-changing SEO landscape with confidence. Embrace the power of the Google Search Console API in Node.js, and let it be your guide to success in the digital world.
Good luck, entrepreneurs, on your SEO adventures, and may your websites soar high in search rankings, attracting the attention of your target audience and driving business growth!